Rector: upgrade PHP application
March 28, 2025In the dynamic world of PHP development, we often find ourselves having to refactor code, update it to newer versions of PHP or adapt to changes in the libraries and frameworks used. Manual modifications can be time-consuming and error-prone, which is where Rector comes in. This tool enables automated code transformation using rules that can be easily configured and extensible. In this article, we'll look at how to install Rector and how to use it effectively in modernizing PHP applications.
The PHP Rector tool is a powerful automated refactoring tool that helps migrate code between PHP versions, updating frameworks and improving code quality. With predefined rules and the ability to create custom transformations, it allows developers to efficiently upgrade code without having to manually edit each file. And that's why it saves time and therefore money.
Typical basic examples of what Rector does for me
Examples of what Rector can convert automatically an newer version, which saves a lot of manual work!
1. Converting old array notation to modern notation (array()
→ []
)
If you are using PHP 5.4 or later, Rector can convert your old array notation to the new one:
Original code:
$array = array(1, 2, 3);
After refactoring:
$array = [1, 2, 3];
2. Convert old constructor methods (__construct
instead of class name)
In PHP 4, constructors used to have the same name as the class, which is deprecated in modern versions of PHP. Rector is can be overridden.
Original code:
class MyClass {
function MyClass() {
echo "Hello";
}
}
After refactoring:
class MyClass {
function __construct() {
echo "Hello";
}
}
3. Replacing deprecated functions
For example, each()
was removed in PHP 8.0, so Rector can replace it with the modern foreach
.
Original code:
while (list($key, $value) = each($array)) {
echo "$key => $value";
}
After refactoring:
foreach ($array as $key => $value) {
echo "$key => $value";
}
4. Adding Stricter Types (PHP 7.4+)
Rector can add type declarations for arguments and return values of methods.
Original code:
function add($a, $b) {
return $a + $b;
}
After refactoring:
function add(int $a, int $b): int {
return $a + $b;
}
5. Convert to ::class
instead of string class names
Modern PHP allows you to use ::class
instead of class names as strings.
Original code:
$className = "MyClass";
$obj = new $className();
After refactoring:
$className = MyClass::class;
$obj = new $className();
These are just basic examples - Rector can make many more modifications, such as automatic code for newer versions of PHP, removing deprecated functions, and rewriting Symfony/Laravel code to recommended standards.
6. Converting from annotations to attributes (Doctrine, Symfony, PHP 8+)
PHP 8 introduced native attributes to replace traditional PHPDoc annotations. Rector ti help you automatically rewrite old annotations into modern attributes.
Original code (with annotations):
use Doctrine\ORM\Mapping as ORM;
/**
* @ORM\Entity
* @ORM\Table(name="users")
*/
class User {
/**
* @ORM\Id
* @ORM\GeneratedValue
* @ORM\Column(type="integer")
*/
private int $id;
/**
* @ORM\Column(type="string", length=255)
*/
private string $name;
}
After refactoring to attributes:
use Doctrine\ORM\Mapping as ORM;
#[ORM\Entity].
#[ORM\Table(name: "users")]
class User {
#[ORM\Id]
#[ORM\GeneratedValue]
#[ORM\Column(type: "integer")]
private int $id;
#[ORM\Column(type: "string", length: 255)]
private string $name;
}
After that, you still need to modify the Doctrine configuration to use attributes instead of annotations and you can try the application to see how it works after changing from annotations to attributes.
Converting with annotations to attributes is the most common task I use Rector for. You can deal with both Doctrine entity conversion and controller annotation in Symfony Framework. Also, if you need help with this feel free to contact me.
For more details on this topic, see How to Upgrade Annotations to Attributes
✅ Other benefits of converting annotations to attributes:
- Shorter and clearer writing
- No dependency on PHPDoc parser required
- Attributes are part of the language, which improves stability and performance
Installing Rector
Rector is opensource and distributed under the free MIT license
We can install Rector within the project, but it can be installed anywhere else - you just need a sufficiently recent version of PHP. So if you have a project with an old version of PHP, you can't install and run Rector within the project, but you have to install it aside and run it with a different newer version of PHP.
Rector can be installed into a project using Composer. It is recommended to install it as dev dependency, as it will usually only be used during development and refactoring.
composer require rector/rector --dev
After installation, you can create a configuration file, for example:
vendor/bin/rector init
This command will generate a basic rector.php
file where you can define refactoring rules.
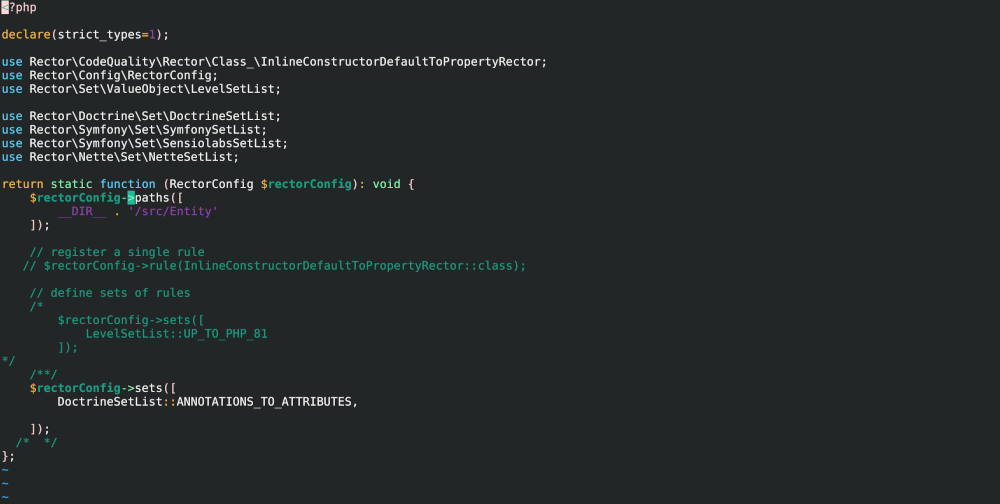
Starting Rector
vendor/bin/rector process src
This command will refactor all PHP files in the src
folder according to the rules set in rector.php
.
Run without applying modifications to the source code
vendor/bin/rector process src --dry-run
Video
A video with a description of Rector and a practical demonstration of how to use Rector to refactor an application.
Articles on a similar topic
How to speed up the web
Go programming language
Analysis of assignment and pricing of software project development
Python program to control Docker using the API
How to use MailCatcher to test emails
Python OpenAI API
Creating a WebSocket web application and setting up a proxy
Project management: agile software development
How to run old PHP applications
What a good programmer should know
Rust programming language
NodeJS: development, server configuration
Nette security bug CVE-2020-15227
REST API: platform API
Custom web and mail hosting with ISP Config software
Programming in SQL: PostgreSQL, MySQL/MariaDB
HTTPS: secure web
NoSQL database Mongo DB
Connecting to Microsoft SQL Server from Linux
What is the job description of a programmer
Python application localization
Which mail and web hosting to choose
Digispark - Program Atmel ATtiny microcontroller with Arduino IDE
Development for ARM processors with Arduino IDE
How to program the ESP8266 WiFi processor
What platform should I choose for my e-shop? For example, Prestashop
OpenStreetMap and GPS routes in the map on the web
Open smartphone with Linux - Openmoko Neo FreeRunner
Newsletter
If you are interested in receiving occasional news by email.
You can register by filling in your email
news subscription.
+